HTTPで通信が出来ると次に要望が来るのがHTTPS通信ですね。
今回はRaspberryPIでHTTPS通信を行います。
まずはopen-sslをインストールする。
wget https://www.openssl.org/source/openssl-1.0.2.tar.gz
tar xvf openssl-1.0.2.tar.gz
cd openssl-1.0.2
./config zlib shared no-ssl2
make && sudo make install ←結構時間が掛かります。
ソース
1 #include <stdio.h>
2 #include <string.h>
3 #include <stdlib.h>
4 #include <unistd.h>
5
6 #include <netdb.h>
7 #include <sys/socket.h>
8 #include <netinet/in.h>
9
10 #include <openssl/ssl.h>
11 #include <openssl/err.h>
12
13 int
14 main(int argc, char **argv)
15 {
16 int sock;
17 struct sockaddr_in server;
18 struct addrinfo hints, *res;
19
20 SSL *ssl;
21 SSL_CTX *ctx;
22
23 char msg[4096];
24
25 if (argc != 4) {
26 printf("https-client host port path ¥n");
27 return -1;
28 }
29 char *host = argv[1];
30 int port = atoi(argv[2]);
31 char *path = argv[3];
32
33 //httpsで通信
34 memset(&hints, 0, sizeof(hints));
35 hints.ai_family = AF_INET;
36 hints.ai_socktype = SOCK_STREAM;
37 char *service = "https";
38
39 //IPアドレスに変換
40 if ((getaddrinfo(host, service, &hints, &res)) != 0) {
41 printf("error getaddrinfo¥n");
42 return -1;
43 }
44
45 //ソケットを確保
46 if ((sock = socket(res->ai_family, res->ai_socktype, res->ai_protocol)) < 0) {
47 printf("error socket¥n");
48 return -1;
49 }
50
51 //接続
52 if (connect(sock, res->ai_addr, res->ai_addrlen) != 0) {
53 printf("error connect¥n");
54 return -1;
55 }
56
57 //SSL初期化して接続
58 SSL_load_error_strings();
59 SSL_library_init();
60 ctx = SSL_CTX_new(SSLv23_client_method()); //SSLの種類 SSLv2,SSLv3,TLSv1のどれかから自動
61 ssl = SSL_new(ctx); //
62 SSL_set_fd(ssl, sock); //ソケットに割り付ける
63 SSL_connect(ssl); //SSL接続
64
65 //GETを送信
66 sprintf(msg, "GET %s HTTP/1.0¥r¥nHost: %s¥r¥n¥r¥n", path, host);
67 SSL_write(ssl, msg, strlen(msg));
68 printf("%s¥n%s¥n", msg, service);
69
70 //応答を読み込む
71 while(1) {
72 char buf[4096];
73 memset(buf, '¥0', sizeof(buf));
74 int read_size;
75 read_size = SSL_read(ssl, buf, sizeof(buf));
76 if(read_size <= 0){
77 break;
78 }
79 printf("%s", buf);
80 }
81
82 //後処理
83 SSL_shutdown(ssl);
84 SSL_free(ssl);
85 SSL_CTX_free(ctx);
86 ERR_free_strings();
87 close(sock);
88
89 return 0;
90 }
コンパイル
cc -g https-client.c -o https-client -L/usr/local/ssl/lib -I/usr/local/ssl/include -lssl -lcrypto
実行結果
千葉県を代表する八千代銀行さんのインターネットバンキングログインページです。
/https-client www.parasol.anser.ne.jp 443 '/ib/index.do?PT=BS&CCT0080=0597' | nkf
GET /ib/index.do?PT=BS&CCT0080=0597 HTTP/1.0
Host: www.parasol.anser.ne.jp
https
HTTP/1.1 200 OK
Date: Sat, 04 Jun 2016 00:23:03 GMT
Server: Apache
Cache-Control: no-cache
Vary: Accept-Encoding
Connection: close
Content-Type: text/html;charset=Windows-31J
<!DOCTYPE html>
<html lang="ja">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=Shift_JIS" />
<meta http-equiv="Content-Style-Type" content="text/css" />
<meta http-equiv="Content-Script-Type" content="text/javascript" />
<meta http-equiv="Pragma" content="no-cache" />
<meta http-equiv="imagetoolbar" content="no" />
<title>八千代ネットバンキング</title>
<link rel="styleSheet" href="/docs/css/jquery.autocomplete.css" type="text/css"><link rel="styleSheet" href="/docs/css/jquery.ui.autocompleteEx.css" type="text/css"><link rel="styleSheet" href="/docs/css/jquery-ui.custom.css" type="text/css"><link rel="styleSheet" href="/docs/css/aaui001.css" type="text/css"><link rel="styleSheet" href="/docs/css/livevalidation_style.css" type="text/css"><link rel="styleSheet" href="/docs/css/0597/style.css" type="text/css"><link rel="styleSheet" href="/docs/css/0597/page.css" type="text/css"><link rel="styleSheet" href="/docs/css/aaui015.css" type="text/css"><link rel="styleSheet" href="/docs/css/0597/frame_topmenu.css" type="text/css"><link rel="styleSheet" href="/docs/css/0597/commonstyle.css" type="text/css"><link rel="styleSheet" href="/docs/css/0597/nav.css" type="text/css"><link rel="styleSheet" href="/docs/css/0597/printstyle.css" type="text/css">
<script language="JavaScript"></script>
長いので省略
</div>
</body>
</html>
pi@raspberrypi:~/program/08.https-client $
GETの結果をファイルにリダイレクトしてIEで表示してみた。
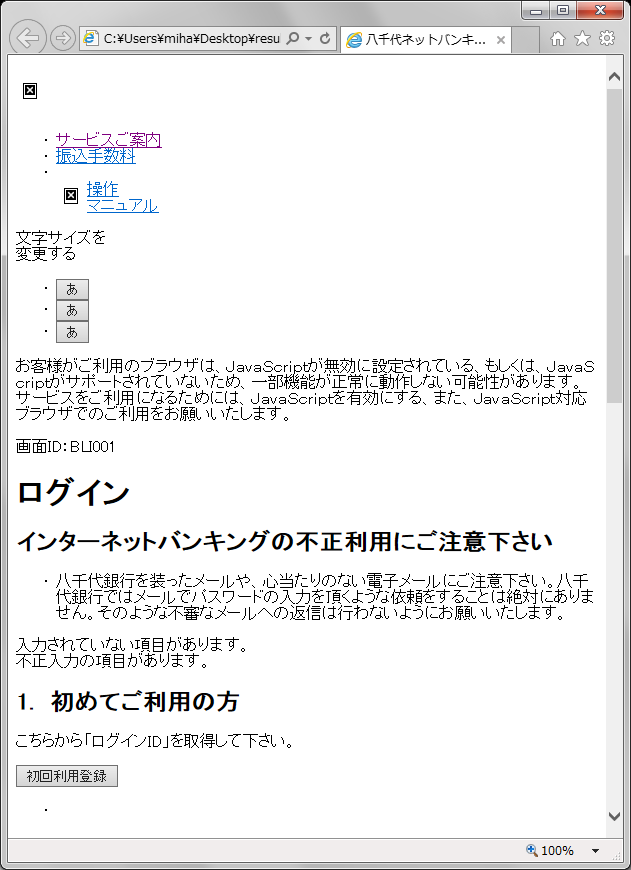
JUGEMテーマ:趣味